Summary: in this tutorial, we will show you how to insert data into a table in an SQLite database using the Java JDBC.
To insert data into a table using the INSERT statement, you use the following steps:
- First, connect to the SQLite database.
- Next, prepare the
INSERT
statement. If you use parameters for the statement, use a question mark (?) for each parameter. - Then, create an instance of the
PreparedStatement
from theConnection
object. - After that, set the corresponding values for each placeholder using the set method of the
PreparedStatement
object such assetInt()
,setString()
, etc. - Finally, call the
executeUpdate()
method of thePreparedStatement
object to execute the statement.
The following program inserts three rows into the table warehouses
that we created in the creating table tutorial.
package net.sqlitetutorial;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
/**
*
* @author sqlitetutorial.net
*/
public class InsertApp {
/**
* Connect to the test.db database
*
* @return the Connection object
*/
private Connection connect() {
// SQLite connection string
String url = "jdbc:sqlite:C://sqlite/db/test.db";
Connection conn = null;
try {
conn = DriverManager.getConnection(url);
} catch (SQLException e) {
System.out.println(e.getMessage());
}
return conn;
}
/**
* Insert a new row into the warehouses table
*
* @param name
* @param capacity
*/
public void insert(String name, double capacity) {
String sql = "INSERT INTO warehouses(name,capacity) VALUES(?,?)";
try (Connection conn = this.connect();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, name);
pstmt.setDouble(2, capacity);
pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
InsertApp app = new InsertApp();
// insert three new rows
app.insert("Raw Materials", 3000);
app.insert("Semifinished Goods", 4000);
app.insert("Finished Goods", 5000);
}
}
Code language: Java (java)
After running the program, you can check the warehouses
table in the test.db
database using the following SELECT statement:
SELECT
id,
name,
capacity
FROM
warehouses;
Code language: SQL (Structured Query Language) (sql)
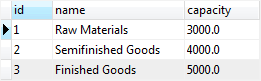
In this tutorial, you have learned how to insert data into a table in the SQLite database from the Java program.
Was this tutorial helpful ?